Nature of Code - Random Walker
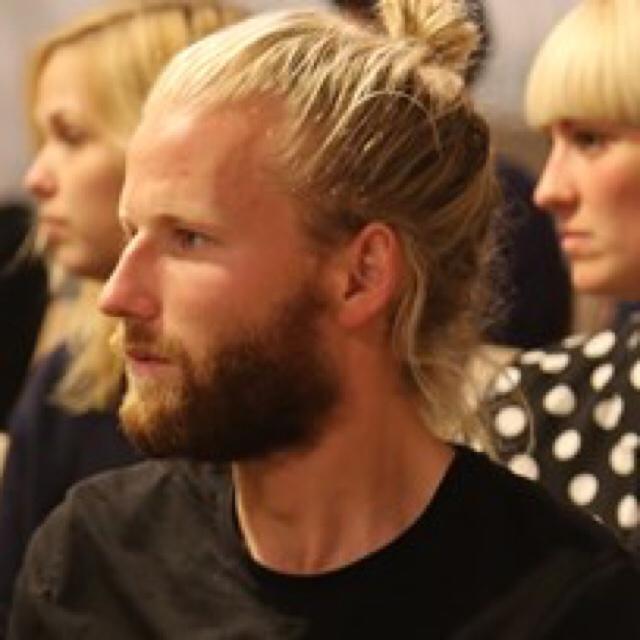
This week we expanded on the random walker concept. We were introduced to different ideas and had a brief introduction to vectors. The assignment for next class was to come up with some different iterations of the random walker sketch.
I didn’t really have any specific random walk sketch in mind for this weeks assignment - so I played around with different basic ideas and variations. These are some of the sketches I ended up with.
The first sketch uses location, velocity and acceleration for movement. In addition to that, the direction is determined using the heading() function which calculates the velocity direction and changes the angle of the object according to that. Randomness in generated by the P5.vector2D() function.
class Walker {
constructor(x, y) {
this.location = createVector(x, y);
this.velocity = createVector(0, 0);
this.acceleration = createVector(0, 0);
}
display() {
var angle = this.velocity.heading();
push();
rectMode(CENTER);
translate(this.location.x, this.location.y)
rotate(angle)
noStroke();
fill(160, 200, 200)
rect(0, 0, 50, 10)
triangle(25, 10, 35, 0, 25, -10);
pop();
}
update() {
var v = p5.Vector.random2D()
v.limit(0.1);
this.location.add(this.velocity);
this.velocity.add(v);
}
edges() {
if (this.location.x & gt; width) {
this.location.x = 0;
}
if (this.location.x & lt; 0) {
this.location.x = width;
}
if (this.location.y & gt; height) {
this.location.y = 0;
}
if (this.location.y & lt; 0) {
this.location.y = height;
}
}
}
The following sketch is using the createShape() function to connect an array of random walkers. A vertex between each walkers’ x and y position turns the array into one structure with movement going in various directions.
let walkers = [];
let totalWalkers = 40;
function setup() {
createCanvas(400, 400);
for (var i = 0; i & lt; totalWalkers; i++) {
walkers.push(new Walker(random(width / 3, width / 3 * 2), random(height / 3, height / 3 * 2)));
}
}
function draw() {
background(220);
//ellipse(width/2,height/2,10,10);
for (var i = 0; i & lt; totalWalkers - 1; i++) {
walkers[i].display();
walkers[i].update();
push();
beginShape(QUADS);
fill(255, 255, 255)
vertex(walkers[i + 1].x + 5, walkers[i + 1].y)
vertex(walkers[i + 1].x - 5, walkers[i + 1].y)
vertex(walkers[i].x - 5, walkers[i].y)
vertex(walkers[i].x + 5, walkers[i].y)
endShape(CLOSE);
pop();
}
}